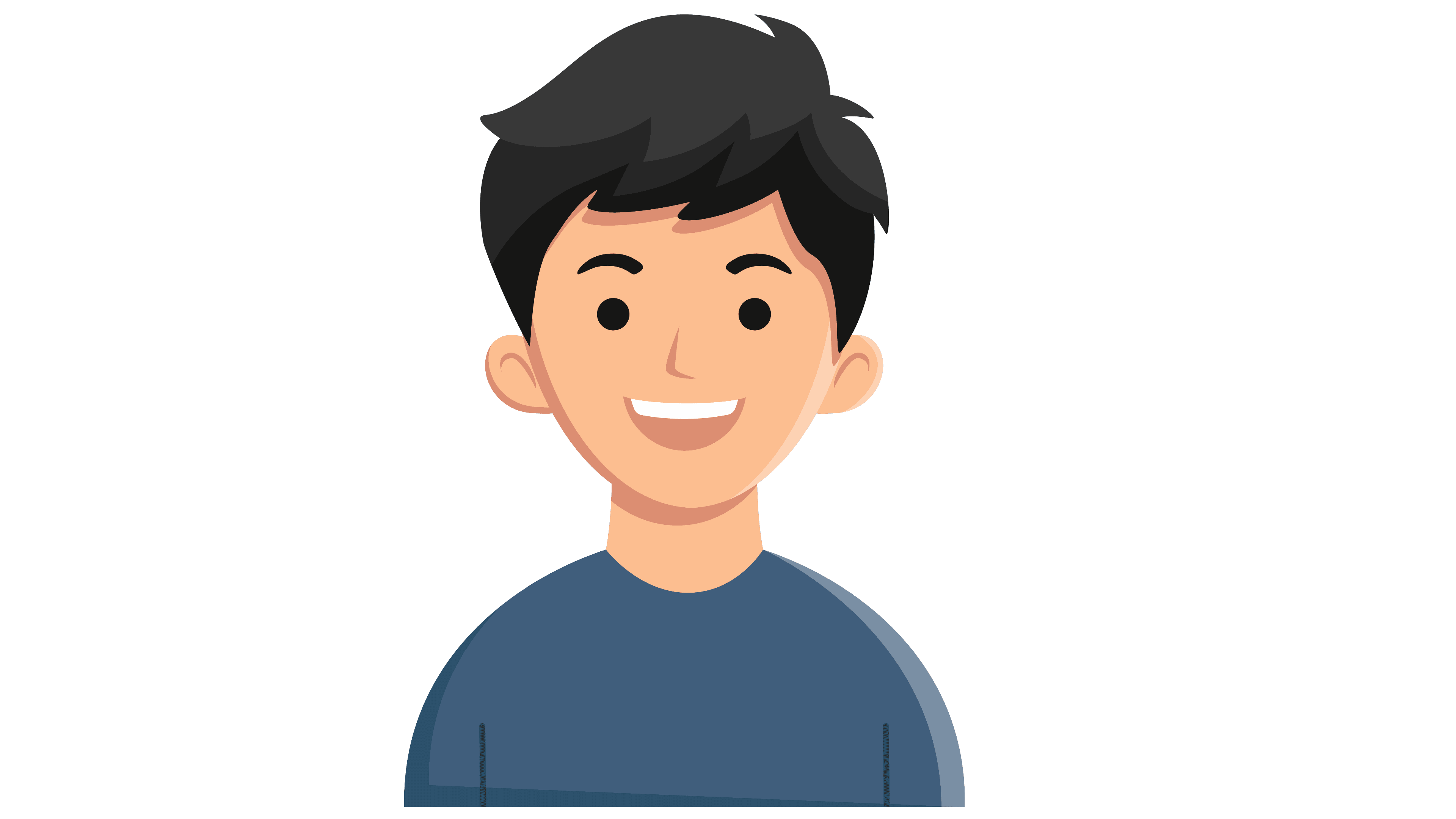
Ken Kariuki
Shiftpulse Marketers
15 Advanced JavaScript and TypeScript Tricks for Experienced Developers
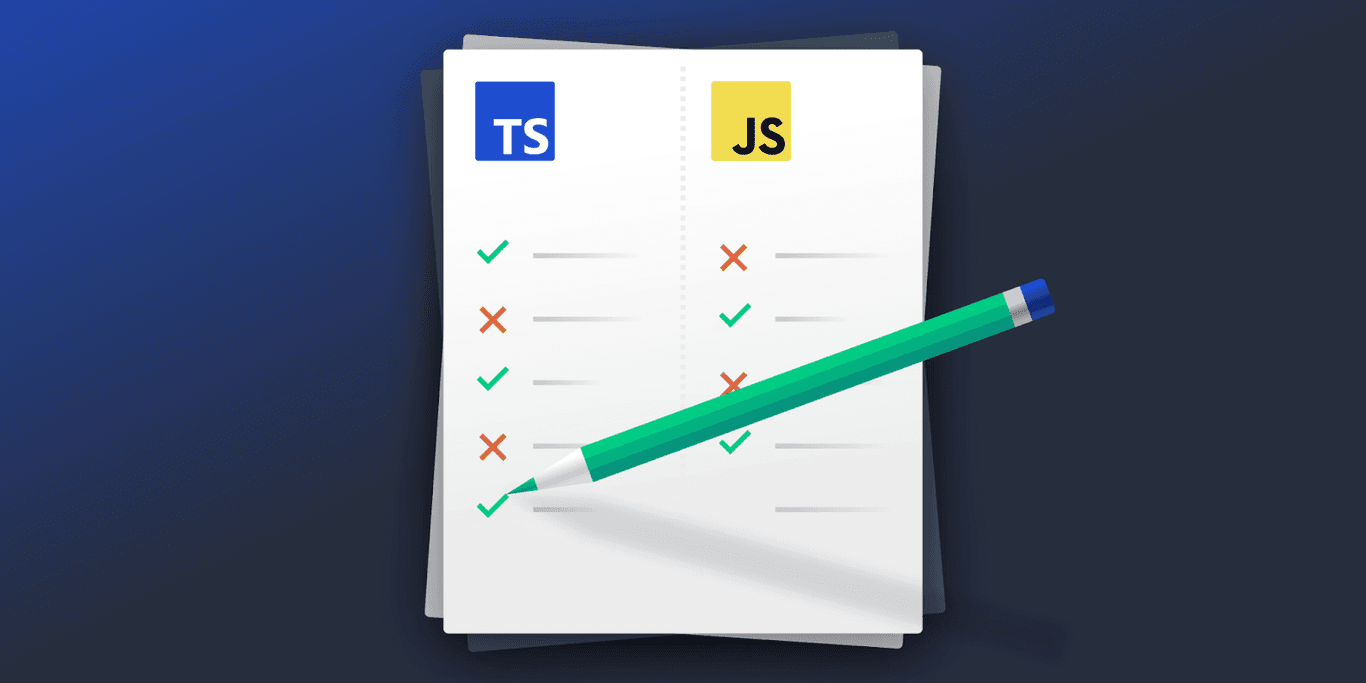
As a seasoned developer, you're likely always looking for ways to enhance your skills and write cleaner, more efficient code. JavaScript and TypeScript, with their ever-evolving ecosystems, offer a plethora of advanced techniques that can significantly elevate your programming prowess.
Here are 15 advanced tricks to help you master these languages:
JavaScript
Mastering Closures: Closures are functions that "remember" their lexical environment, even after the outer function has finished executing. This allows for data encapsulation and the creation of private variables, enhancing code organization and security. JavaScript
function outerFunction(x) {
let privateVar = x;
return function innerFunction(y) {
return privateVar + y;
};
}
Harnessing the Power of Generators: Generators are special functions that can be paused and resumed, allowing for efficient handling of asynchronous operations and infinite data streams. JavaScript
function* fibonacci() {
let = [0, 1];
while (true) {
yield curr;
= [curr, prev + curr];
}
}
Embracing Functional Programming with Currying: Currying transforms a function with multiple arguments into a sequence of functions, each taking a single argument. This technique promotes code reusability and composability. JavaScript
const add = (x) => (y) => x + y;
const addFive = add(5);
console.log(addFive(3)); // Output: 8
Optimizing Performance with Memoization: Memoization caches the results of expensive function calls, preventing redundant computations and boosting performance. JavaScript
function memoize(fn) {
const cache = {};
return function(...args) {
const key = JSON.stringify(args);
if (cache) {
return cache;
}
const result = fn(...args);
cache = result;
return result;
};
}
Leveraging the Proxy Object for Metaprogramming: The Proxy object allows you to intercept and customize operations performed on an object, enabling metaprogramming and advanced behavior manipulation. JavaScript
const handler = {
get: function(target, prop) {
return prop in target ? target : 37;
}
};
const p = new Proxy({}, handler);
p.a = 1;
p.b = undefined;
console.log(p.a, p.b); // Output: 1 37
Implementing Asynchronous Queues: Asynchronous queues help manage and process asynchronous tasks in a controlled manner, preventing race conditions and ensuring orderly execution.
Utilizing Advanced String and Number Methods: Explore lesser-known string and number methods like padStart, padEnd, toLocaleString, and isInteger to streamline string manipulation and number formatting.
Exploring the Intl Object for Internationalization: The Intl object provides powerful tools for formatting dates, numbers, and currencies according to different locales, facilitating internationalization efforts.
Diving Deep into Regular Expressions: Master advanced regular expression techniques like lookaheads, lookbehinds, and named capture groups for precise string matching and manipulation.
TypeScript
Creating Custom Utility Types: Extend TypeScript's built-in utility types like Partial, Required, and Readonly to create custom types tailored to your specific needs.
Implementing Type Guards for Enhanced Type Safety: Type guards are functions that narrow down the type of a variable within a conditional block, improving code clarity and type safety. TypeScript
function isString(value: any): value is string {
return typeof value === 'string';
}
Leveraging Conditional Types for Dynamic Typing: Conditional types allow you to express types that depend on a condition, enabling dynamic type derivation and increased code flexibility. TypeScript
type IsNumber<T> = T extends number ? 'yes' : 'no';
type TypeA = IsNumber<number>; // 'yes'
type TypeB = IsNumber<string>; // 'no'
Working with Indexed Access Types for Flexible Data Structures: Indexed access types allow you to access types within other types using dynamic keys, enabling type-safe manipulation of complex data structures.
Utilizing Template Literal Types for String-Based Type Constraints: Template literal types combine string literals and type manipulation to create types that adhere to specific string patterns.
Exploring Advanced Type Inference Techniques: Deepen your understanding of TypeScript's type inference mechanisms to write less verbose code while maintaining strong type safety.
By mastering these advanced JavaScript and TypeScript tricks, you'll not only enhance your coding skills but also unlock new levels of efficiency, maintainability, and expressiveness in your projects.